Assignment 2 (due 7 March)
(last updated: 3 March 2001)
In this assigment you will write an object oriented program using Java
that will consist of a class hierarchy of shapes (e.g., square, triangle,
circle) that implement a shape interface. The shape interface requires
that a shape provides instance methods to reveal its name, and its perimeter
and area measurements.
To be handed in: a diskette labeled with your name, containing
the subdirectories comprising your JBuilder project subtree (your .jpr
project file should be in the root of the subtree) and printouts of your
Java source files (*.java). Please place your diskette and printouts
in a large envelope also labeled with your name.
The project will consist of the following interface and classes:
-
Instance methods:
String
name()
returns the name of the shape (e.g., "Triangle")
double
area()
returns the area measurement of the shape
double
perimeter()
returns the perimeter measurement of the shape
-
Abstract class name:
-
Instance attributes:
-
Constructor:
-
Instance methods:
//must
have these, since this class implements Shape interface;
//but
leave abstract...
abstract
String name();
abstract
double area();
abstract
double perimeter();
//Using
methods from Shape interface, override toString() method
//--that's
the whole reason for this abstract class!
String
toString()
returns
a reference to an object of class String that contains a string representation
of what the shape looks like, e.g., "Rectangle with perimeter 14 and area
12" -- this method should use the Shape interface methods to construct
such a string...
-
Instance attributes:
double radius; //
the length of the circle's radius
-
Constructor:
constructs
a new Circle object with the specified radius
Sample invocation:
Circle aCircle = new Circle(3.0);
-
Class name:
-
Instance attributes:
double
sideA,
sideB, sideC; // the lengths of the three sides of the triangle
-
Constructor:
Triangle(double
a, double b, double c)
constructs a new Triangle object with the three specified side lengths
Sample
invocation:
Triangle aTriangle = new Triangle(3.0, 4.0, 5.0);
-
Instance methods:
must
implement the three methods left abstract by abstract class AbstractShape.
Use Heron's formula to calculate the area of a triangle given the lengths
of its three sides:
area = Math.sqrt( s * (s - sideA) * (s - sideB) * (s - sideC) ),
where
s = (sideA + sideB + sideC) / 2
-
Abstract class name:
-
Instance attributes:
double
length,
width; // the length and width of a rectangle
-
Constructor:
by
definition, abstract classes don't have constructors
-
Instance methods:
must
implement the three methods left abstract by abstract class AbstractShape.
Recall the formulae to calculate the area and perimeter of a rectangle:
-
Class name:
-
Instance attributes:
-
Constructor:
//
constructor takes two parameters to initialize
//
corresponding inherited instance attributes
MyRectangle(double
l, double w)
Sample
invocation:
MyRectangle
aRectangle = new MyRectangle(4.0, 6.0);
-
Class name:
ShapeApplet
extends
JApplet // once again, your user interface Is-a JApplet!
-
Instance attributes:
Vector
shapes;
//
a collection of shapes
Notice
how your ShapeApplet JApplet uses (contains) shape objects instantiated
from the previous classes.
-
Instance methods:
You'll
define methods corresponding to the instances of JButtons on your JApplet
panel. The buttons will add circles, triangles, rectangles, and squares
to the shapes vector. The parameters
for
these new shapes will be taken from the corresponding JTextFields on the
applet panel. Similar to the previous programming assignment, you'll
need to parse the double values from the strings returned by getText().
You'll find the following static (class) method helpful:
Double.parseDouble(s) -- returns a double
value parsed from its String parameter.
A
"List Shapes" button will use an object of class Iterator to iterate through
the
shapes vector, and use System.out.println() to list the stored
shapes.
Unlike the previous assignment, the objects stored in the shapes
vector can be instances of different shape classes -- but all the
shape classes extend class AbstractShape, which implements the Shape
interface. Since AbstractShape overrides the toString() method for
shape objects, we can cast references from our iterator's .next() method
to be (AbstractShape) references, and printing our shapes is as
simple as: System.out.println( aShape ); !!!
(!) Note: All constructors and all instance methods should
be public, and all attributes should be protected.
The GUI (Graphical User Interface) should look something like this:
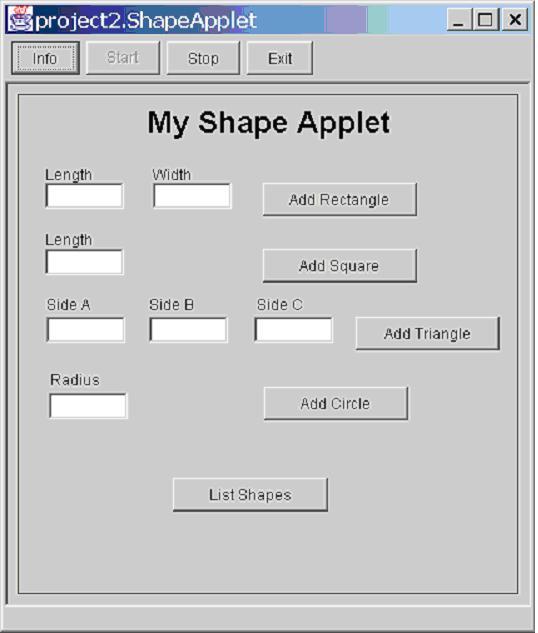
The output from the System.out.println()'s should look something like
this:
Shape Rectangle with area = 20.0 and perimeter
= 18.0
Shape Square with area = 36.0 and perimeter = 24.0
Shape Triangle with area = 6.0 and perimeter = 12.0
Shape Circle with area = 113.09733552923255 and perimeter = 37.69911184307752