COP3402
Systems Programming
Fall 2011
|
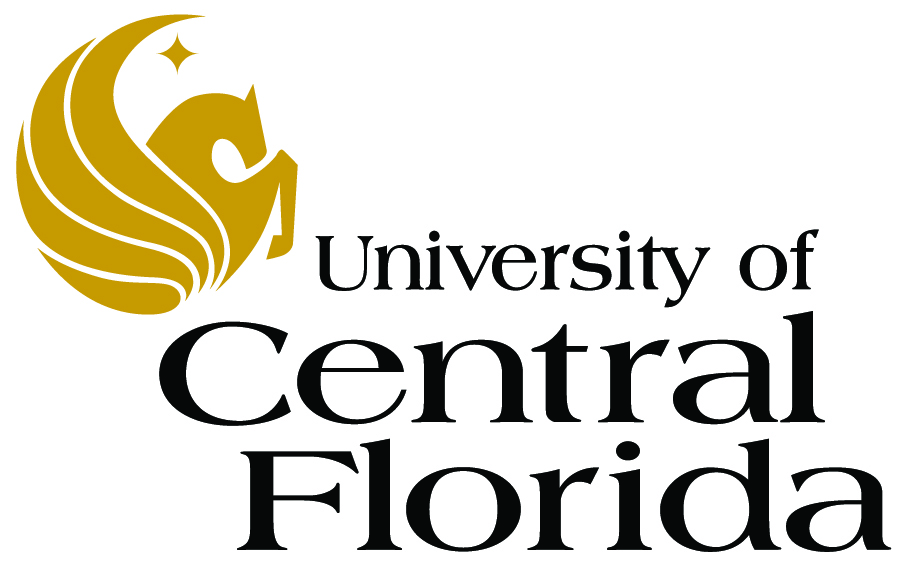
email:
charles.e.hughes@knights.ucf.edu Use Subject COP3402
Lectures: MW 1930-2045 (7:30PM to 8:45PM), CL1-320;
29 class periods, each 75 minutes long.
Labs: All on Wednesday in COMM-111; Section 11: 4:30-5:20; Section 12: 5:30-6:20; Section 13: 6:30-7:20
Go To Week 1, 2, 3, 4,
5, 6, 7,
8, 9, 10,
11, 12, 13,
14, 15
Instructor: Charles Hughes; Harris Engineering Center HEC-247C;
823-2762 (use e-mail; I spend most of my time in my lab)
Office Hours: MW 9:45AM-10:45AM; M 5:30-6:30; and by appointment
GTA: Steven Zittrower, steven.zittrower@gmail.com, HEC-250; Th 3:00 to 5:00
Wenhui Li, liwenhui6328@hotmail.com, HEC-254; Tuesday 4:00 to 5:00
Text: Compilers:
Principles, Techniques, & Tools, Second Edition by Alfred V.
Aho,
Monica S. Lam, Ravi Sethi,
and Jeffrey D. Ullman (DRAGON)
ISBN-10: 0321486811 | ISBN-13: 9780321486813
We will cover Chapter 1-6, 8, 9 of DRAGON book
Prerequisites: COP3502 (Computer Science I)
Web Pages:
Base URL: http://www.eecs.ucf.edu/courses/cop3402/fall2011
Course Home Page: COP3402Fall2011
Notes Folder: Notes
Assignments Folder: Assignments
Code Samples: CodeSamples
Course Wiki: http://mclserver.eecs.ucf.edu/trac/courses/wiki/COP3402Fall2011
Assignments: 5 to 8, one of which is the major project. Each assignment will have a due date
and 10% will be subtracted for each day late (up to 2 days late, 20%
off; more than two days late
results in no credit)
Exams: One midterm and a final
Material: I will draw heavily from text by
Aho, Lam, Sethi and Ullman. You are responsible
for
material discussed in notes and in in-class discussions. Not all of
this is addressed in text. I highly recommend
attending class, interacting with me and listening very carefully when
I say a topic is important to me; hint, hint about exam questions ;-)
Important Dates: Labor Day -- September 5; Midterm Exam -- October 10 (Tentative);
Withdraw Deadline -- October 27; Final -- December 7, 7:00PM-9:50PM
Evaluation (Tentative):
Mid Term -- 20%
Final Exam -- 30%
Programming and Other Assignments -- 20-25%
Final Programming Project -- 20-25%
Wild Card -- 5% (used to increase weight of what you did well on)
Grading
will be A >= 90%, B+ >= 87%, B >=
80%, C+ >= 77%, C >= 70%, D >= 60%, F < 60%. (minuses may be used in some cases)
Week#1: (8/22, 8/24) -- Chapter 1; Syllabus , Dragon Chapter 1 slides, Hughes Week 1/2 slides
- Goals, expectations and rules of the course
- A bit of an insight into what I
do and what my background is in this domain
- Language Processors: pre-processors, compilers,
interpreters and analyzers
- Source to Target: Compile, [Assemble,] Link, Load ([...] means optional)
- Target can be machine language for native or virtual machine
- A JVM (Java Virtual Machine) interprets bytecodes but Java runtime systems usually include a JIT (just-in-time translator) to translate to native code
- Microsoft's equivalent virtual machine is the CLR (Common Language Runtime) which interprets CIL (Common Intermediate Language) and/or produces native code
- The father and mother of these virtual machine codes were the Pascal P-code and the Smalltalk bytecode
- source
-> Preprocessor ->
modified source -> Compiler ->assembly program -> Assembler
-> relocatable code -> Linker/Loader -> target code
- Linker/Loader also has input from libraries and previously compiled/assembled code
- Phases of a compiler
- character stream -> Lexical analyzer -> token stream
- token stream -> Syntax analyzer -> syntax tree
- syntax tree -> Semantic analyzer (Context Analysis/Type Checking) -> syntax tree
- syntax tree -> Intermediate
code generator -> intermediate representation
- intermediate representation -> Machine independent code optimizer (improver) -> intermediate representation
- intermediate representation -> Code generation -> target machine code
- target machine code -> Machine dependent code optimizer (improver) -> target machine code
- Symbol table is key data structure used throughout process
Week#2: (8/29, 8/31)
-- Chapter 1; Dragon Chapter 1 slides, Hughes Week 1/2 slides
- Overviews and simple examples of phases
- Lexical analysis
- Syntactic analysis
- Semantic Analysis
- Intermediate
code generation
- Code optimization (improvement)
- Code generation
- Synbol table management
- Grouping of phases in passes (a pass reads a stream or structure and procudes another stream of structure)
- Front-end vs back end phases and the value of virtual traget machines
- Compiler tools
- scan generator (lex and flex)
- parser generator (yacc and bison)
- syntax-directed translator (convert tree to intermediate code)
- automatic code generator (translation rules from intermediate to target code)
- data flow analyzer (aid code optimzation by analyzing def-use chains, etc.)
- compiler construction toolkits (collections of above tools)
- A little history of programming languages and their translations -- influence of theory
- Memory hierarchies and optimization
- Lexical analysis -- hand crafter
Assignment #1
Description of Lexical Analysis Assignment
Due: Monday, September 19 before the end of day (11:59PM)

Week#3: (9/7) -- Chapter 2; Dragon Chapter 2 slides, Hughes Week 3/4 slides
- A little history of programming languages and their translations -- influence of theory
- Grammars and languages
- Determinism vs non-determinism
- Ambiguity and its relation to
non-determinism
- Non-ambiguity in expressions:
associativity and precedence
- Grammars for various programming
constructs: Expressions
- Expression grammars (precedence
and associativity)

Week#4: (9/12, 9/14)
-- Chapter 2; Dragon Chapter 2 slides, Hughes Week 3/4 slides
- The Chomsky hierarchy and the hierarchy of recognizers
- Syntax-directed translation
- Context free grammars
- Parse trees
- Notion of push down automata
(stack memory)
- Determinism vs non-determinism
- Ambiguity and its relation to
non-determinism
- Non-ambiguity in expressions:
associativity and precedence
- Grammars for various programming
constructs: Expressions
- Expression grammars (precedence
and associativity)
- Syntax directed translation:
attributes and program fragments (semantic actions)
- Synthesized versus inherited
atributes
- Simple syntax-directed
translation amenable to automated parser generation
- Left vs right recursion and
top-down (predictive) vs bottom-up parsing
- Virtual non-stack machine overview (no explicit registers)
- Virtual stack machine overview: p-code (also no explicit registers)
Assignment #2
Due: Monday, October 3 before the end of day (11:59PM)

Week#5: (9/19, 9/21)
-- Chapter 3; Dragon Chapter 3 slides, Hughes Week 5/6 slides
- Handcrafted lexical analyzer
- Symbol tables (Envirment Blocks)
- Regular
Expressions
- Building a lexical analyzer through
regular expressions
- FLEX lexical analyzer generator
(look for download specific to your platform)
- History of finite state automata
(FSA) -- circuits and pattern matching
- Regular expressions and FSAs
- FSAs and Regular Grammars
- Deterministic vs non
deterministic FSAs
- Syntax analysis
- Top down vs bottom up parsing
- Converting left to right
recursion, maintaining semantic actions
- Left factoring
- Extended BNF
Week#6: (9/26, 9/28) -- Chapter 4; Dragon Chapter 4 slides, Hughes Week 5/6 slides
- Extended BNF
- Syntax graphs / Railroad charts
- Designing a recursive descent parser from syntax graphs
- Predictive parsing (First and
Follow sets)
- Nullable symbols and effect on
First and Follow
- Parsing table from First and
Follow
- Top down vs bottom up parsing
- CKY parsing (great example of
dynamic programming)
Week#7: (10/3, 10/5) -- Chapter 4; Review in Class; Help Sessions in Lab; Hughes Week 7/8 slides
- Reiteration of basic conflicts on top down and
on bottom up parsing
- Stack process for each
- Topics and Promises for
MidTerm # 1
- Sample Exam
-- Complete this for
discussion on 10/5 (key)
- Review and go over sample questions
Week#8: (10/10 (Midterm), 10/12) -- Chapter 4; Hughes Week 7/8 slides
- Midterm
- Symbol tables (again)
- Bottom
up vs top down and leftmost versus rightmost (once again)
- Finite state automata and
pushdown automata
- Bottom up parsing with a PDA
- Lexical, syntactic and
intermediate code based on flex and
bison
- Notions of error recovery in
both various types of parsers
- While language processor:
while.l and while.y
- Bottom up parsing of While
language using Bison
- Intermediate code representation
as triples
Week#9: (10/17, 10/19) -- Chapter 4, Hughes Week 9/10 Slides
- Finite state automata and
pushdown automata (again)
- Non-deterministic parsing via
PDAs
- Symbol table management for
While language
- Symbol table management and hash
coding
- Symbol tables for nested
declarations
- Comments on recursive descent parsing of While
langauge
- Error recovery in recursive
descent
- Initial discussion of data flow
analysis
- LR Parsing
- SLR as first example
- Midterm Exam#1 Key
- Note: 10/27 is the
Withdraw Deadline
Week#10: (10/24, 10/26) -- Chapter 4; WITHDRAW DATE IS THURSDAY, OCT. 27, Hughes Week 9/10 Slides
- LR Parsing
- SLR as first example
- Basic algorithm
- LR(0) items and sets of items
- Closure of sets of items
- Action and Goto functions
- Building the parser
- LR(1) items and sets of items
- Closure of sets of items
- Action and Goto functions
- Building the canonical LR parser
- Merging states and the LALR
parser
- Reduce/reduce errors that might
arise
- Building the LALR parser
Assignment #3
Due: Monday, November 7 by 11:59PM

Week#11: (10/31, 11/2) --Chapters 4, 5 and 6 of DRAGON, Hughes Week 11/12 slides
- Ambiguity
- Error recovery
- Discussion of Assignment#3
- Parse trees versus syntax trees
- Syntax directed translation
- Attributes (synthesized vs
inherited)
- S-attributed and L-attributed
parse trees
- Side effects during translation
- Creating syntax trees via
attributes
- Code generation
- Triples versus Quads (compact
versus easy to move)
- Indirect triples as a compromise
Week#12: (11/7, 11/9)
-- Chapters 5 and 6 of DRAGON, Hughes Week 11/12 slides
- Project Discussions -- lots of them
- Back to recursive descent
- A bit of language basics
- static versus dynamic
- parameter passing
- Project Discussions
Project
Due: 12/1 at 11:59PM
Week#13: (11/14, 11/16) -- Chapter 8; Material
comes from project description and Reference Solution, Hughes Week 13/14 slides
- Interpreter folder Assignments/Projects/Triples Interpreter
- Project Discussions -- lots of
them
- Sample recursive descent compiler for Pascal-S (Niklaus Wirth)
- Handling of function returns
- Handling of Case
-- Jump tables versus hashed jump tables vs if--then
- Peephole optimizations
Week#14: (11/21, 11/23) -- Chapter 9, Hughes Week 13/14 slides
- Data flow analysis
- Inter- vs Intra-procedural analysis
- Forward vs Backward flow
- May vs Must
- Def-Use chaining
- Variety of optimizations and analyses based of data flow

Week#15: (11/28, 11/30) -- Review
in Class; Help Sessions in Lab
- Final Exam Topics
- Structure of Final
- Sample Final
(Key)
Final Exam; Wednesday, December 7; 7:00PM - 9:50PM in CL1-320

Week#16: (12/5, 112/7) -- Help Sessions
in HEC-101
- Help Sessions (Practice Problems with Answers)
Final Exam; Wednesday, December 7; 7:00PM - 9:50PM in CL1-320
© UCF (Charles E. Hughes)
-- Last Modified December 5, 2011