Distributed Applications
in the Enterprise
Fall 2002 |
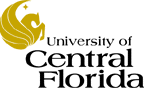
email:
ceh@cs.ucf.edu
Structure: MW 16:00-17:15, CL1 109;
29 class periods, each 75 minutes long.
Go To Week 1, 2,
3,
4,
5,
6,
7,
8,
9,
10,
11,
12,
13,
14,
15,
16
Instructor: Charles Hughes; CSB 206; 823-2762; ceh@cs.ucf.edu
Office Hours: MW 1:30-3:00
GTA: Mathew Lowery; CC1 202; mlowery@cs.ucf.edu
Office Hours: T 3:30-5:00; F 1:30-3:00
Text: Deitel, Deitel and Santry, Advanced Java™ 2 Platform,
Prentice-Hall, 2002.
Prerequisites: COP3330 (OOP), COP3503 (CS2), EEL 4882 (OS),
CGS 2545 (Databases).
Implementation Environments: You will be regularly using Java.
You do not need to be a Java expert, but you must be familiar with it at
the level of student who has completed COP3330 and COP3503. Feel free to
ask others for guidance to quickly solve trivial problems with Java syntax
or with using Borland JBuilder and the JDK. If you have serious problems,
come see me or the GTA, Mathew Lowery.
Assignments: 3 or 4 small to moderate programming assignments
(some are multi-part) using a variety of frameworks and APIs. Around 5
or 6 non-programming assignments. One fairly large project.
Exams: Midterm and a final, and maybe a quiz.
Material: From Deitel: Chapters 1-3, 6-10, 12-16, 22-24, 26-29,
Appendix A, B, C. Parts of this are on CD in text. Class notes.
Important Dates: Labor Day -- September 2; MidTerm -- October
2; Withdraw Deadline -- October 11; Veterans Day -- November 11; Final
-- December 4, 16:00-18:50
Evaluation (Tentative):
Mid Term -- 100 points
Final Exam -- 125 Points
Non Programming Assignments -- 25 Points
Regular Programming Assignments -- 75 Points
Project -- 125 Points
Bonus1 -- 25 Points (increase in points in exam where you did better)
Bonus2 -- 25 points (increase in points in weight of better of exams
or assignments)
Total Available: 500
Grading will be A>=90%, B>=87%, B>=80%, C>=77%, C>=70%, D>=50%,
F< 50%
Resources:
Free Software IDEs: JBuilder
7 Personal; Forte
for Java CE; DrJava (for a
refresher on Java); Java
Tutorial
Sites: Deitel&Deitel; Sun
Java Site; Apache; Lots more in text
Weeks#1: (8/19, 8/21) -- Chapter 1 & Notes
-
Syllabus
-
Philosophy and Goals
-
Rules of the game
-
Unless explicitly stated otherwise, all assignments (programming and non-programming)
must be your own independent work. Copied or cooperatively developed materials
will be rejected, leading to no grade on the assignments.
-
Cheating on exams will lead to immediate expulsion from the course.
-
All mail sent to me that is associated with this course MUST have
COP4610 in its subject field.
-
The schedule below is highly speculative. In fact, I already know I will
change the order of material to accommodate discussing those topics in
time to be useful for your projects.
-
The number of chapters covered may seem daunting, but many of them get
their size due to the embedded examples. In some cases, the entire meat
of the chapter is only a few pages long.
-
Concurrency: benefits and challenges
-
Threads
-
Java Support for Concurrency
-
Threads : either inherit from Thread class or implement Runnable interface
-
constructor in Runnable specifies object that provides code for thread
-
constructor can also specify a string to identify thread
-
EOSort thread example. Run it.
See
it.
-
Synchronize : specifies critical section using an object as lock
-
can do at granularity of method
-
can do at granularity of a block
-
Locks are reentrant
-
Locks can be temporarily given up : wait and notify
-
Distributed applications
-
Paradigms
-
Channels (send/receive)
-
Distributed objects (RPC/RMI)
-
Middleware agent (space/message queue)
-
Support for various paradigms in Java
Assignment #1:
Read the example browser pane in Figure 2.1 of Deitel. There
is a problem with their code. It occurs in "boundary conditions." In fact,
most common algorithm design errors occur at the boundaries (like at the
base case in an inductive argument). You must find the problem(s). Turn
in a one-page write-up (an e-mailed document) with your description of
the problem(s) and your suggested solution(s). This must be transmitted
prior to Monday's class. You may not discuss this with any of your classmates,
friends, relatives or local congressional staff. I want to see what you
can deduce on your own.
Due: August 26
Programming Assignment #1:
The Threads example provides
code to do an even-odd transposition sort. EOSort is a multithreaded approach
that works because each thread maintains control of the single CPU once
it completes the sleep at the top of its run loop. Add simple yields or
additional sleeps to EOSort that cause it to change active threads. If
you do this right, the consequence is that EOSort occasionally fails to
carry out the sort properly. This will be trivial at the code end, but
takes some thought to figure out how you might get the sort to fail without
changing its basic logic.
Turn in a zip file with your project (be sure that the zip keeps all
directory structures) and a write-up. Your write-up must discuss your placement
of sleeps or yields and the consequences of these changes. Submit to the
GTA by e-mail (mlowery@cs.ucf.edu).
Be sure to include your name and COP4610 as part of the subject field in
your e-mail.
FAQs
Due: September 11
Week#2: (8/26, 8/28) --
Chapter
2 & Chapter 3
-
Distributed applications
-
XML
-
Patterns
-
Prototype
-
Bridge
-
Iterator
-
Memento
-
Strategy
-
Concurrency (single threaded, balking, read/write lock, two phase termination)
-
Command
-
Swing classes
-
Java's Event Model
-
listeners
-
single queue
-
why threads are spawned
-
Deitel Web Browser
-
Containers
-
Command Design
-
Action interface, Action Event
-
HyperlinkListener interface
-
Multiple Document Interface (MDI)
-
Drag and Drop (DnD)
-
gesture
-
operation
-
Transferable interface
-
DropTagetListener interface
-
Internationalization
-
Accessibility (Java Access Bridge)
-
Model View Controller (MVC) Framework (Architecture)
-
Observer design pattern
-
Observer interface
-
Observable class
-
Delegation
Programming Assignment #2:
Exercise 2.4 from text: Create an image
viewer that supports drag-and-drop loading of images.
Turn in a zip file with your project (be sure that the zip keeps all
directory structures). Submit to the GTA by e-mail (mlowery@cs.ucf.edu).
Be sure to include your name and COP4610 as part of the subject field in
your e-mail.
FAQs
Due: September 23
Week#3: (9/4) -- Chapter
6
Labor Day on 9/2
-
Beans
-
Concept
-
component-based application construction
-
Components as building blocks; component assembly tools
-
VRML events as an example
-
Serializable requirement
-
depth-first graph traversal
-
avoiding the cycle trap
-
Introspection
-
the reflection API (java.lang.reflect)
-
Bean design pattern
-
Properties (for propertyName)
-
public Datatype getPropertyName ()
-
public isPropertyName() method for boolean properties
-
public void setPropertyName(Datatype data)
-
Indexed properties
-
public Datatype[] getPropertyName ()
-
public Datatype getPropertyName (int index)
-
public void setPropertyName(Datatype[] data)
-
public void setPropertyName(int index, Datatype data)
-
Custom events
-
Customizing a bean (component)
Week#4: (9/9, 9/11) -- Chapter
6
-
Beans (we will need to use Forte4Java for this material)
-
Using Beans
-
Custom events
-
Customizing a bean (component)
-
BeanInfo interface
-
expose limited features or those that do not follow bean design pattern
-
Property editor (constraining a property's values)
Week#5: (9/16, 9/18) -- Chapter
7
-
Help session on building Java applications and visual interfaces using
JBuilder 7
-
Security -- General overview
-
Fundamental properties
-
privacy
-
integrity
-
authentication
-
non-repudiation
-
History
-
Symmetric/secret key
-
Asymmetric/public key
-
Cryptanalysis
-
Key agreement prtrocols
-
Key management
-
JCE
-
Password-based encoding
-
Use of decorator pattern
-
Digital Signatures
-
Hash functions
-
SHA-1 (160 bit digest)
-
MD5 (128 bit digest)
-
Supports integrity but not non-repudiation
-
timstamping can limit repudiation
-
Certificate authorities and authentication
-
Secure Socket Layer (SSL)
-
Decorates TCP/IP communication
-
Supports option authentication
-
server authentication is common
-
client authenication is rare
-
Negotiated session keys
-
JSSE
-
Secure class communication, loading and interaction in Java
-
Bytecode verifier
-
Class loaders and namespaces
-
Policy files
Week#6: (9/23, 9/25) -- Chapter
8, Notes
-
Databases
-
Review of relational operators
-
tables represent relationships among fields
-
rows or records
-
columns or fields or attributes
-
primary key (column whose values uniquely identify a row) -- example is
author incremented id #
-
Rule of Entity Integrity says every record must have a unique primary key
value
-
ER diagrams are used to visually describe table relationships
-
Types of relationship (1-1, 1-many, many-1, many-many)
-
foreign keys are fields in one that that are primary keys in another (used
in joins)
-
SQL is Simple Query Languge for operating on relational databases
-
selection
-
SELECT * FROM employees // get all records
-
SELECT lastName, firstName from employees // just the names
-
selection criteria
-
SELECT fields FROM table WHERE criteria
-
operators are <,>,<=,>=,=,<>,LIKE
-
LIKE is for pattern matching (% is any string, _ is any character)
-
WHERE lastName LIKE 'H_G% matches HUGHES, HUG, HAG, HUGE, HIGH, HAGAR,
etc.
-
SOME SQL implementations use * in place of %
-
ORDER BY used to sapecify order, e.g., ORDER BY lastName ASC // low to
high
-
JOIN operation
-
SELECT fields FROM table1,table2 WHERE tables1.field=table2.field
-
SELECT fields FROM table1,table2 WHERE field1=field2
-
some SQL implementations support direct specification of natural join (one
common field)
-
INSERT INTO
-
INSERT INTO table [(fields)] VALUES (values)
-
UPDATE SET
-
UPDATE table SET field1=value1, ... WHERE criteria
-
DELETE FROM
-
DELETE FROM table WHERE criteria
-
Optimization of relational queries (in Notes,
not in text)
-
JDBC
-
Protocols
-
JDBC to ODBC -- Microsoft Open Database Connectivity
-
Native API to partly Java -- uses JNI to access native API
-
JDBC to net pure Java -- translates to network protocol (non DB specific)
-
Native to protocol pure Java -- translates to DB specific network protocol
-
Last two protocols are preferred
-
The process
-
get a Connection -- may involve name, password
-
create a Statement (or several)
-
execute a query -- returns a ResultSet
-
if necessary, get ResultMetaData
-
can tell you number of columns, column classes, etc.
-
process resultSet using next() method (must start with next())
-
can access columns by name or number (faster)
-
PreparedStatement
-
maintains statement in compiled form (fast execution)
-
can have parameters that are set prior to statement execution (uses ? to
specify and parameter number to set)
-
Transactions
-
transaction makes a series of SQL statements appear to be atomic
-
auto commit -- close commits
-
commit or rollback before close
-
ACID
-
Atomicity - The entire sequence of actions must be either completed or
aborted. The transaction cannot be partially successful.
-
Consistency - The transaction takes the resources from one consistent state
to another.
-
Isolation - A transaction's effect is not visible to other transactions
until the transaction is committed.
-
Durability - Changes made by the committed transaction are permanent and
must survive system failure
-
The above properties are critical to transaction semantics
-
CallableStatement
-
Stored Procedures in database
-
Batch processing
-
Updatable ResultSet properties (if available)
-
scrollable means can move cursor forward or backward
-
sensitive means the result set is updated as changes are made
-
if insensitive, you must requery database to upadte result set
-
concurrency -- if updatabale then updates to result set are immediately
reflected in database
-
Topics and Promises for
MidTerm
-
Samples for MidTerm
Assignment #2:
At end of Database
Notes
Due: September 30
Week#7: (9/30, 10/2)
-
Review, answers to sample
questions for MidTerm
MidTerm on 10/2
Week#8: (10/7, 10/9) -- Chapter
9 & Chapter 10
-
Servlets
-
Architecture
-
Get/Post
-
Session Tracking
-
Multi-tiered applications
-
JSP
-
Review of Exam
Assignment #3
The latest issue of the Communications of the ACM is all about
component assembly (Beans, in Java terminology). Everyone must read the
Introductory paper on pages 30-34. You must then read two of the other
papers in this sepecial section, excluding the last paper (page 83) and
the one that starts on page 41. You must follow up on one reference in
one of these two papers. You must then write a 5 to 10 page paper that
both presents what you read and provides your personal insights. The paper
must be in 12 point Times Roman or Times font, double-spaced, and have
margins of one inch on all sides. All references must appear at the end
using the same standards as the CACM articles. You must include a header
on each page, except the first, with your name, the page number, and the
title of your paper (abbreviated if necessary). Submit the paper electronically
to me and to Mat.
Due: October 28
Note: Last Day to Withdraw is 10/11
Week#9: (10/14, 10/16) -- Chapter
10
-
JSP
-
Beans assignment
-
Project discussion (MeasureMe example)
Programming Assignment #3:
This is a very simple Beans assignment, involving just Swing
classes, which are themselves Beans. You must create a user interface that
has one JTextField, two JButtons and a JComboBox. Typing in a value and
pressing return in the text field should trigger a change to the first
button label to match the text just typed. Pressing this button should
cause the button's label to be added to the items in the combo box. The
button should be labelled "CLEAR". Pressing it removes all items from the
combo box. This must be accomplished using BeanBuilder's
visual design tool. Submit the xml file generated by BeanBuilder electronically
to Mat (mlowery@cs.ucf.edu).
Due: November 4
Project Assignment
Project FAQs
Week#10: (10/21, 10/23) -- Chapter
13
-
Distributed paradigms (full
version)
-
Remote Method Invocation
-
Serializing (marshaling) objects / persistency
-
Distributed Objects:
-
Creating a service:
Compile code producing skeleton (server side) and stub
(client side)
Note: Usually involves IDL (Interface Definition
Language)
-
Server action:
Register service with Object Manager (sometimes just registry
on server)
Note: Object manager is often called an ORB
(object request broker)
-
Client action:
Request service from Object Manager
Note: This usually involves a Name Server
-
Object manager action:
Negotiate with service providers for an object
Note: This sometimes involves Reflection
Deliver remote object to client
-
Client/Server Interaction:
Client uses remote object to get services
Server receives remote messages, provides results
Arguments and results can be
by-value (local copy) or
by reference (remote handle)
This exchange involves serializing/unserializing objects
Note: Serializing is sometimes called marshalling
-
Roles are reversed when server sends messages to remote objects from client
-
The above is standard whether Java RMI or CORBA or whatever
-
Java's Solution: RMI
-
RMI IDL is Java Interface; rmic compiles skeleton and stub
Write interface which must extend Remote interface
Generate skeleton and stubs
-
Server Application:
Write service implementation corresponding to interface
Server registers a service by name on some RMI port
Server must start an RMI registry prior to
this
Note: default port is 1099
-
Client Applet:
Write client applet
Client "looks up" service
Note: Need server IP address, port, and name
of service
Lookup returns remote object
Note: Class of remote object is name of Interface
(stub)
-
RMI Examples (zip format)
-
Building and installing servlets
-
Required libraries for compilation (servlet jar)
-
Placement in Tomcat area
-
Managing Tomcat container
-
Project Discussion
Programming Assignment #4:
The RMI Bid Example (Mike's one) has
a few problems. This was actually an early version that he did. You are
to find all problems (Hint: Try to accept a bid; Hint: Is it fair for the
one who offers an item for sale to kick up the bid?). Fix the user interface.
In particular, how do you exit gracefully?
Make-up: If you want to improve a grade on an
old assignment, you can expand this assignment by changing it to work within
the Activation framework. The second example in Chapter 13 describes this.
Submit the entire project directory (maintaining its structure) electronically
to Mat (mlowery@cs.ucf.edu) as
a single zip file.
FAQs
Due: November 20
Week#11: (10/28, 10/30) -- Chapter
14, Chapter 15, Chapter
16 & Chapter 23
-
Enterprise Java Beans (EJBs)
-
Container
-
load balancing
-
serializing unused beans
-
LRU strategy
-
Access is through container (no retention or passing of handle to object
-
JNDI to register and find bean by name
-
Work is offloaded from software development to bean deployment
-
Session versus Entity Bean
-
Session Beans
-
Stateful vs stateless
-
stateless do not need to be serialized and can be used by multiple clients
-
stateful can hold attributes across an entire session
-
Remote interface (inherits from EJBObject)
-
accessors to bean attributes and services
-
Home interface (inherits from EJBHome)
-
create (and perhaps remove, find)
-
create specifies remote interface type as returned bean type
-
Implementation does not declare that it implements either interface
-
the connection is made when EJB is deployed
-
Examples from text
-
Entity Beans
-
Relation to database row
-
Bean managed versus container managed persistence
-
Also has Remote and Home interfaces
-
Home interface must include a findByPrimaryKey, as well as create
-
Examples from text
-
Message Queues
-
Tuple Spaces
Week#12: (11/4, 11/6) --Chapter
22 & Chapter 23
-
Object Request Broker
-
Brief discussion of CORBA
-
Jini
-
Discovery
-
Join
-
Lookup
-
Group and/or attributes
-
Remote or local proxy
-
Distributed garbage collection (also issue in RMI)
-
JavaSpaces
-
Transient vs persistent space
-
Operations
-
notify
-
read, readIfExists
-
take, takeIfExists
-
write
-
Pure space has eval and no predicate operations
-
Examples
-
Provides communication and coordination
-
Semaphores
-
Arrays
-
Embarrassingly parallel problems
-
image manipulation
-
LOD computation
-
Lightweight communicating agents
-
Efficient management of space
-
Leases
-
Used extensively in Javaspaces
-
Prevents deserted tuples from cluttering space
-
Can renew
-
Often used in JavaSpaces to create a pseudo predicate read/take
-
Earth Echoes
-
Wednesday will be devoted to meetings with project teams
-
I will meet with teams in 15 minute intervals from 1:30 to 5:30 in CSB
206
-
Schedule your time slot to get the best for you
-
Mat will clean up on Friday from 1:30 to 3:00
Optional Assignment:
Using space notation (notify, read, take, write), present the
design of a system that supports location-based storytelling, as in the
Earth Echoes system discussed in class. You may invent your own ways by
which information is deposited and acccessed, so long as location and genre
are the key means of organization/retrieval. This is a design document
from which others should be able to implement the system using JavaSpaces,
so it must be detailed in use/case studies and the essential organization
of the repository. The document must be in word or rtf format. You can
scan in sketches if you don't have a decent other way to show visual aspects
of your design. The expected size of the document is between 3 and 5 pages,
including figures. Submit the design document electronically to me (ceh@cs.ucf.edu)
and to Mat (mlowery@cs.ucf.edu).
This assignment may be used to offset the damage from some previous
assignment that you missed or did poorly on.
Due: December 3
Week#13: (11/13) -- Chapter
21
-
Mixed Reality -- a peek into my research program
-
Overheads
from ACM Lecture
Veterans Day on 11/11
Week#14: (11/18, 11/20) -- Chapter
26, Chapter 27 & Chapter
28
-
CORBA
-
IDL (agreements on types and services)
-
Real time version exists (TAO)
-
Java and Corba
-
Peer-to-peer computing
-
General Concepts
-
Dynamic collaboration
-
Using resources at the edges of the Internet
-
Distributed computation (SETI)
-
Mobile agents
-
Reduction of bottlenecks
-
Limitations on central cataloging
-
Distributed search
-
Finding all or some nodes
-
Parallel operation
-
Time to live (TTL)
-
Anonymity
-
Pure versus hybrid P2P systems
-
JXTAPlatform and language independence
-
Peer groups
-
Advertisements (XML right now)
-
Peer discovery (peer is subject, not object)
-
LAN-based (limited horizon)
-
Cascaded (peers in my horizon use peers in theirs)
-
Rendezvous (pseudo server for peers)
-
Firewalls (bounce in or out through trusted peer)
Week#15: (11/25, 11/27)-- Chapter
28 & Chapter 29
- Outline for Project Reports
- Peer-to-peer
- SOAP
- Attacks on network services
- Final Exam Topics and Promises
- Sample Final Exam Questions
- By popular demand, Wednesday's class will be optional
Week#16: (12/2)
-
Discussion and Return of Assignments
-
Review for Final Exam
-
Sample Final Exam questions
-
Final Exam Topics
Final Exam:
-
Wednesday December 4; 16:00 - 18:50
© Charles E. Hughes, ceh@cs.ucf.edu -- Last Modified
November 26, 2002